Backtracking, Unit Testing and Tilesets
- cmcrter
- Dec 11, 2021
- 3 min read
Backtracking
In the project, I'm only planning to implement the backtracking in the simplest sense, returning to the initial state of the grid. This means that the process will start completely again if there's no possible tile for a cell. Although that wasn't too difficult to set up, it is worth talking about the different ways of backtracking and when they're used.
When Paul Merrell released their 180~ page dissertation on Model Synthesis, backtracking is discussed with multiple aspects of fixing the problem in mind. The initial fix in section 3.3.6 is to revert the model back to the point of failure and retrying. But not retrying from the one block, but having the overall model split into smaller sections, and retrying with the smaller section made.
I saw a similar approach on this tweet by Oskar Stålberg, where it looks like a local chunk of the Wave Function Collapse is reset (highlighted in red). And this may be the other way to look at doing in the future, since it doesn't rely on the program knowing where the contradiction started.
Unit Testing
For the base functionality of the project, I want to make sure it is working exactly as intended. To do this, I've written some unit tests using NUnit in the unit testing framework in the unity package manager.
What a unit test looks like
Here are the first 2 unit tests I set up, the rest follow the same pattern. There's the Arrange section where I make the classes, the act section where I'll do something with them then the assert section where the checks of the test are.
private Grid ExampleGridCreation()
{
Grid gridToReturn = new Grid(3, 3);
return gridToReturn;
}
[Test]
public void NeighboursTest()
{
Grid gridToUse = ExampleGridCreation();
//Getting the neighbours of the centre cell, the grid should be 0, 1, 2
//3, 4, 5
//6, 7, 8
//So the x and y would be 1 and 1, since they start from 0
Tuple<List<Cell>, List<Vector2>> CentreNeighbourhood = gridToUse.GetNeighbours(1, 1);
Tuple<List<Cell>, List<Vector2>> TopLeftNeighbourhood = gridToUse.GetNeighbours(0, 0);
Tuple<List<Cell>, List<Vector2>> TopRightNeighbourhood = gridToUse.GetNeighbours(2, 0);
Tuple<List<Cell>, List<Vector2>> BottomLeftNeighbourhood = gridToUse.GetNeighbours(0, 2);
Tuple<List<Cell>, List<Vector2>> BottomRightNeighbourhood = gridToUse.GetNeighbours(2, 2);
Assert.AreEqual(CentreNeighbourhood.Item1.Count, 4);
Assert.AreEqual(TopLeftNeighbourhood.Item1.Count, 2);
Assert.AreEqual(TopRightNeighbourhood.Item1.Count, 2);
Assert.AreEqual(BottomLeftNeighbourhood.Item1.Count, 2);
Assert.AreEqual(BottomRightNeighbourhood.Item1.Count, 2);
}
[Test]
public void CoordinatesTest()
{
Grid gridToUse = ExampleGridCreation();
Assert.AreEqual(gridToUse.GridCells[0, 0].CellX, 0);
Assert.AreEqual(gridToUse.GridCells[0, 0].CellY, 0);
Assert.AreEqual(gridToUse.GridCells[2, 2].CellX, 2);
Assert.AreEqual(gridToUse.GridCells[2, 2].CellY, 2);
}
Tilesets
The current testing tile set is good for testing simple rules, however the final project will not be using it. Here are 2 other tilesets I made that can work, although they may take a few tries:
This used a few smaller input models, 2 which are river tiles forming a circle around a tile. Then 2 which are straight lines of river that are capped off at both ends (one horizontal and one vertical). having more grass in it, and decreasing the chances of cap, may make a good park section.
I then moved onto to see if a city section was going to be visually correct. Since I currently only have 3 residential tiles setup, I worked on that first. It's more rarer to be correct than the river input models. However, to me it's visually more appealing.
Combining these tilesets / input models, although dirt could connect to most of the tiles in the tilesets, it's very rare that it all connects correctly. When it does produce a correct grid, most of the time it looks like this:
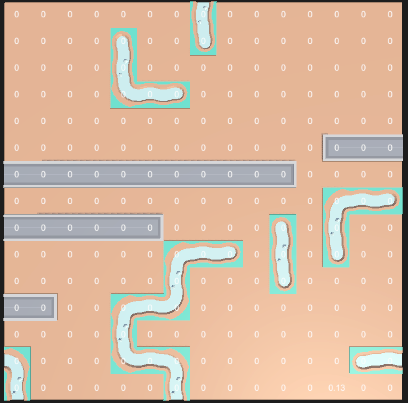
This clearly isn't the wanted goal of combining the tilesets, which is why I had already considered the fix. I referenced adding separate areas in the first blog post, which will use the roads to separate the areas out. This means they probably will not have dead ends on them and the weighting on the straight lines will be increased.
Comments